Use Case - Send files for translation
This page describes the methods you would use to send files for translation into a Codyt project. This can be used for translating a CMS or any other content.
Find project or create new project
Send files for translation into a project
Poll for the completion of workflows / jobs
Optional creation of translated files (if not down by the translation team or automation)
Download translated files
We discuss these steps:
Authenticate
Start with authentication. You need the API 1 end point, see here.
For the authentication method see Connect using GET
curl --location '{{API1URL}}/api/connect?account={{PLATFORMID}}&pwd={{API1PWD}}&json=true'
{{API1URL}}
: For example:https://api.eu.wordbee-translator.com:32570
{{PLATFORMID}}
: Typically your company name. It also shows in the URL when you login to your Wordbee Translator platform.{{API1PWD}}
: Your API 1 password.
The method returns the token that you need to include in all subsequent API calls.
Find project by reference
Typically, you would create a new project in your Wordbee platform and then send content to this project for translation.
Each project is assigned a unique number and you need to find this number by our project’s reference. Below we search for the project named “My Project 1”:
curl --location '{{API1URL}}/projects?token={{token}}&from=0&count=10&reference=My Project 1'
For more details see List or find projects . You can filter by most of the project properties.
The method returns a JSON array with your project. The project ID is named ProjectId
.
To find projects created after a specific date you can use:
curl --location '{{API1URL}}/projects?token=e85e7ab94cdb4c95b8329ba5e7ceab00&filter=CreationDate%3EDateTime(2023%2C%2004%2C%2025)'
Create a new project
You can also create a new project using Create project . It is recommended to create a project as a “clone” of a template project.
Let’s say you created a reference project named “My template” with all the workflows and memories you need. First find the project ID using the method explained above.
Then create a new project using:
curl --location --request POST '{{API1URL}}/projects?token=e85e7ab94cdb4c95b8329ba5e7ceab00&projecttype=1&templateprojectid=5938&reference=My new project'
Upload a file to translate
Now we have a project with its project ID. We also note down the project’s source and target languages. Now we are ready to send a file into this project.
We use this method: Upload new document or new document version . It does basically everything: It uploads our file, it marks the file for translation, it does the word count, it creates jobs and can even assign workers.
Below we upload a web page named “content103.html”. We set the source language to “en” and expect translations into “fr” and “es”. The workflow shall be the project default workflow.
curl --location --request PUT '{{API1URL}}/projects/5938/documents/codytdocument2?token=e85e7ab94cdb4c95b8329ba5e7ceab00&name=content103.html&sourcelocale=en&targetlocales=fr%2C%20de&parserdomain=HTML&workflow=ProjectDefault' \
--header 'Content-Type: application/octet-stream' \
--data '@/C:/My files/content103.html'
Note: URL parameters should always be URL encoded, replace blanks by %20 etc.
In Postman the parameters are shown as:
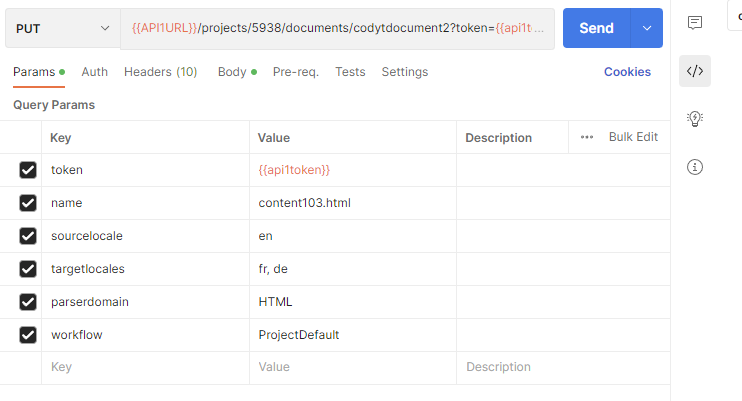
The headers are:
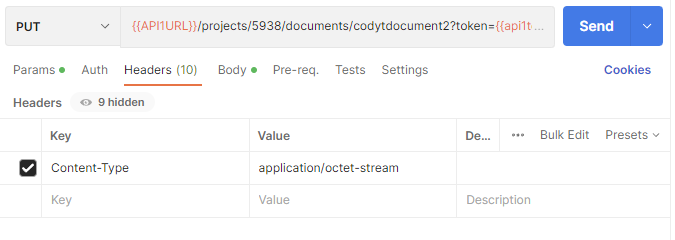
And the actual file is attached as follows:
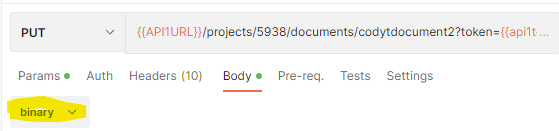
Review in Wordbee Translator
Your file will show up in the documents in the project:

Our test project defines a very simple workflow comprising a single Translation step. Hence we will see 2 jobs: One for French and one for Spanish:
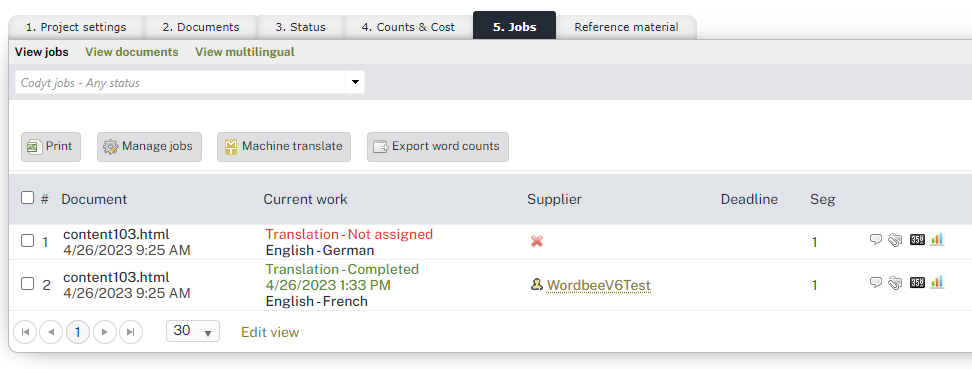
You specify in the project’s workflow profile whether jobs shall be automatically assigned to groups of suppliers. You also specify if multiple workflow steps shall be created (such as Translation + Revision).
Note that in our screenshot above, the French translator already finished the work. So, we should be able to fetch the French translation but not yet the Spanish one.
Find completed workflows
Now that we started sending content for translation, we definitely want to check in to see if any work has been completed! If so, we want to download that work.
Use the “workflows” method to list all completed workflows / documents in a project:
curl --location '{{API1URL}}/projects/workflows?token={{token}}&sourcelocale=en&workflowstatus=1&statusdatefrom=2023-04-25&projectid=5938&from=0&count=100'
The above enumerates all workflows satisfying these conditions:
Check for workflows with English source locale (en)
Check only completed workflows: workflowstatus = 1
Check for project with ID = 5938
Get the first 100 completed workflows / documents (see from / count)
Get only workflows that were completed after the last time we checked (see statusdatefrom). This condition is really useful as it prevents that you get workflows completed in the past (and which you already had processed).
The method returns all workflows (a specific document for a specific source and target locale). Example:
[
{
"BeeDocumentId": 20630,
"BeeDocumentSetId": 6837,
"Comments": "",
"IsCurrent": true,
"LocaleSource": "en",
"LocaleTarget": "en",
"Name": "content103.html",
"OpenTasks": 0,
"ProjectId": 5938,
"ProjectReference": "Stephan API1 Test",
"RevisionSetId": 11319,
"Status_": 1,
"StatusUpdateDate": "/Date(1682486757650+0200)/",
"Version": "",
"VersionDate": "/Date(1682486757610+0200)/"
},
{
"BeeDocumentId": 20630,
"BeeDocumentSetId": 6837,
"Comments": "",
"IsCurrent": true,
"LocaleSource": "en",
"LocaleTarget": "fr",
"Name": "content103.html",
"OpenTasks": 0,
"ProjectId": 5938,
"ProjectReference": "Stephan API1 Test",
"RevisionSetId": 11319,
"Status_": 1,
"StatusUpdateDate": "/Date(1682501618297+0200)/",
"Version": "",
"VersionDate": "/Date(1682486757610+0200)/"
}
]
The first item is the source document itself, we can disregard it.
The second item is the completed French translation. We did see the completed job in the Wordbee platform - see the screenshot further up.
With the target language and the document name, we can now proceed with the download of this translation.
Note: We use present method since we only want to download a translation when all workflow steps are completed.
Build translated file (optional)
In most cases, your project workflow automatically creates the translated file upon completion of a workflow. This can be configured in the Workflow & Supplier page of the project.
Alternatively, you may ask the supplier (in the last workflow step to do so).
Or, finally, you may create the translated file with the API. Just make sure that the workflow is completed (you use the method in the previous chapter).
To create the translated file you run:
curl --location --request POST '{{API1URL}}/projects/5938/files/en/file/translation?token={{token}}&name=content103.html&targetlocale=fr'
You need to specify:
The source locale (see “en”)
The project ID (5938 in our example)
The name of the file to download
The target locale
Download translated file
Download the file using:
curl --location '{{API1URL}}/projects/5938/files/fr/file?token={{token}}&names=content103.html'
You need to specify:
The source locale (see “en”)
The project ID (5938 in our example)
The name of the file to download
The target locale
See Download files or folders for more details.
File names and folders
A file name can include folders such as:
folder1\folder2\myfile.html
Make sure to URL encode the \ in the parameters (replace \ by %5C
)
folder1%5Cfolder2%5Cmyfile.html
Next steps
These are just the most important methods that you likely would use for an integration.
There are many more advanced methods. Check out the present API 1 documentation.